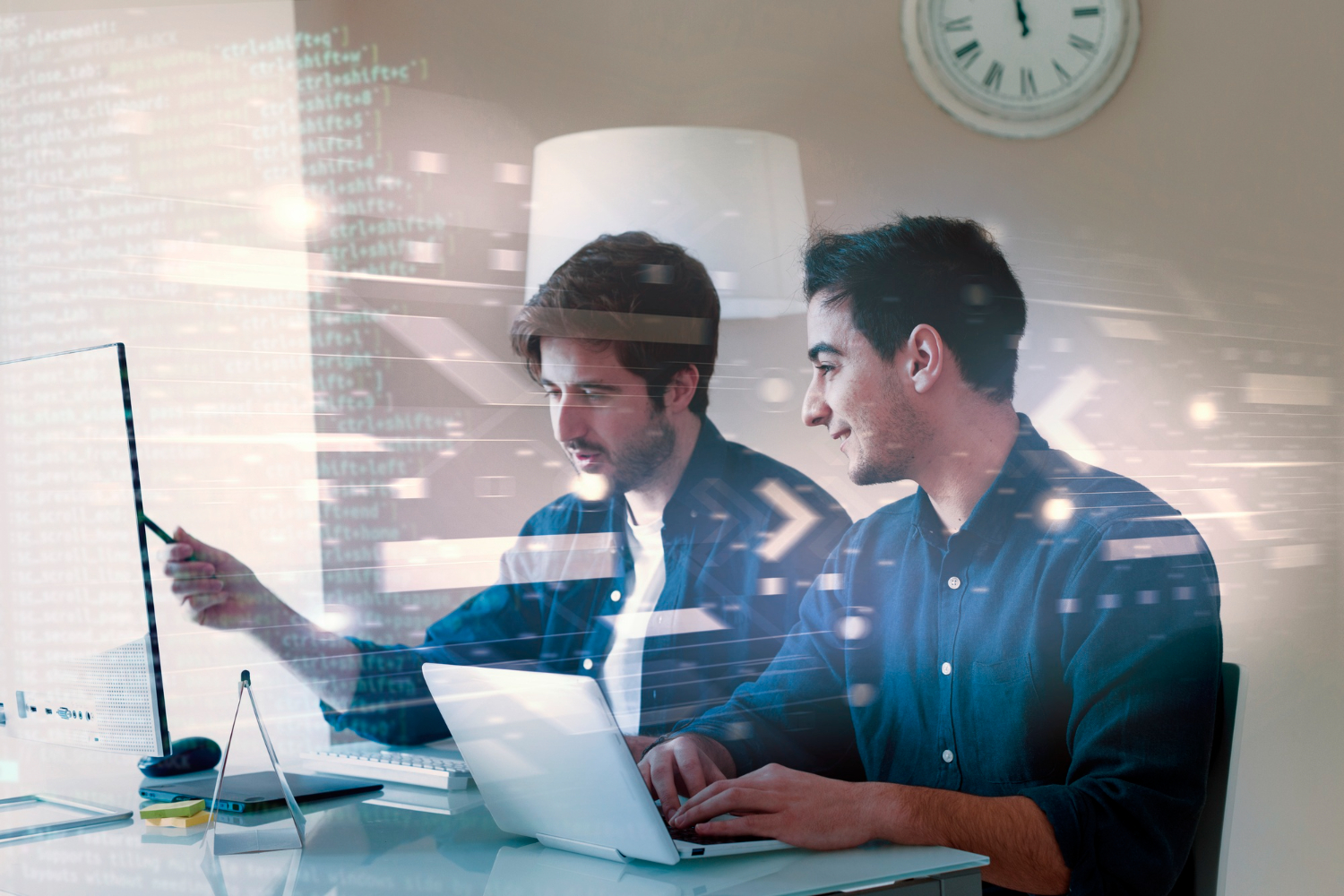
Frontend Development Online Training
Course Description
The Comprehensive Frontend Development Training is a dynamic and immersive online program designed to equip participants with the essential skills and knowledge needed to excel in the rapidly evolving field of frontend development. This comprehensive course covers a broad spectrum of topics, ranging from foundational web technologies to advanced frontend frameworks and industry best practices.
Review each module to ensure a solid understanding, and join this course to start building your frontend development skills today!
Module 1: Introduction to Frontend Development
Getting Started
- Introduction to web development
- Overview of frontend development
- Setting up a development environment (text editor, browser developer tools)
Module 2: Web Technologies Basics
HTML, CSS, and JavaScript Fundamentals
- HTML5 basics: structure, elements, attributes
- CSS3 basics: selectors, properties, styling
- JavaScript basics: variables, data types, operators
- DOM (Document Object Model) manipulation: selecting, modifying elements
Module 3: Responsive Web Design
Responsive Layouts
- Understanding the importance of responsive design
- Media queries and viewport settings
- Implementing fluid grids and responsive design principles
Advanced CSS Layout Techniques
- Flexbox: layout model, properties
- CSS Grid: grid layout, properties
- Building responsive components and layouts
Module 4: Advanced HTML and CSS
Semantic HTML and Accessibility
- Proper use of HTML tags for semantics
- Accessibility best practices and considerations
CSS Preprocessors and Frameworks
- Introduction to SASS or LESS
- Using and customising Bootstrap or Tailwind CSS
Module 5: JavaScript Fundamentals
Basics of JavaScript
- Variables, data types, and operators
- Control structures: if statements, loops
DOM Manipulation with JavaScript
- Selecting and modifying DOM elements
- Event handling and delegation
Module 6: Intermediate JavaScript
Asynchronous JavaScript
- Callbacks, Promises, and Async/Await
- AJAX and Fetch API for data retrieval
ES6+ Features
- Arrow functions, template literals, destructuring
- Let, const, and block-scoped variables
Module 7: Frontend Frameworks
Introduction to Frameworks
- Overview of React, Angular, and Vue
- Pros and cons of each framework
React.js Basics
- Components and props
- State management with Hooks
Module 8: Advanced React.js
React.js Continued
- Routing and form handling
- State lifting and context API
Project Workshops
- Hands-on projects applying React.js concepts
Module 9: Version Control and Collaboration
Git Basics
- Cloning repositories, branching, and merging
- GitHub/GitLab collaboration and version control best practices
Build Tools and Task Runners
- Introduction to Webpack, Babel, npm scripts
- Automating repetitive tasks in the development workflow
Module 10: Testing and Debugging
Testing Fundamentals
- Unit testing with Jest or Mocha
- End-to-end testing with tools like Cypress
Debugging Techniques
- Using browser developer tools
- Debugging JavaScript and CSS issues
Module 11: Final Project and Portfolio Development
Capstone Project
- Building a complete frontend project
- Implementing best practices learned throughout the course
Portfolio Development
- Showcasing projects and skills
- Creating an online portfolio using HTML, CSS, and possibly a static site generator like Gatsby
Module 12: Additional Considerations
Industry Best Practices
- Code reviews, coding standards, and documentation
- Introduction to agile methodologies in frontend development
Continuous Learning
- Resources for staying updated in the fast-evolving frontend landscape
- Encouraging participation in online communities and forums