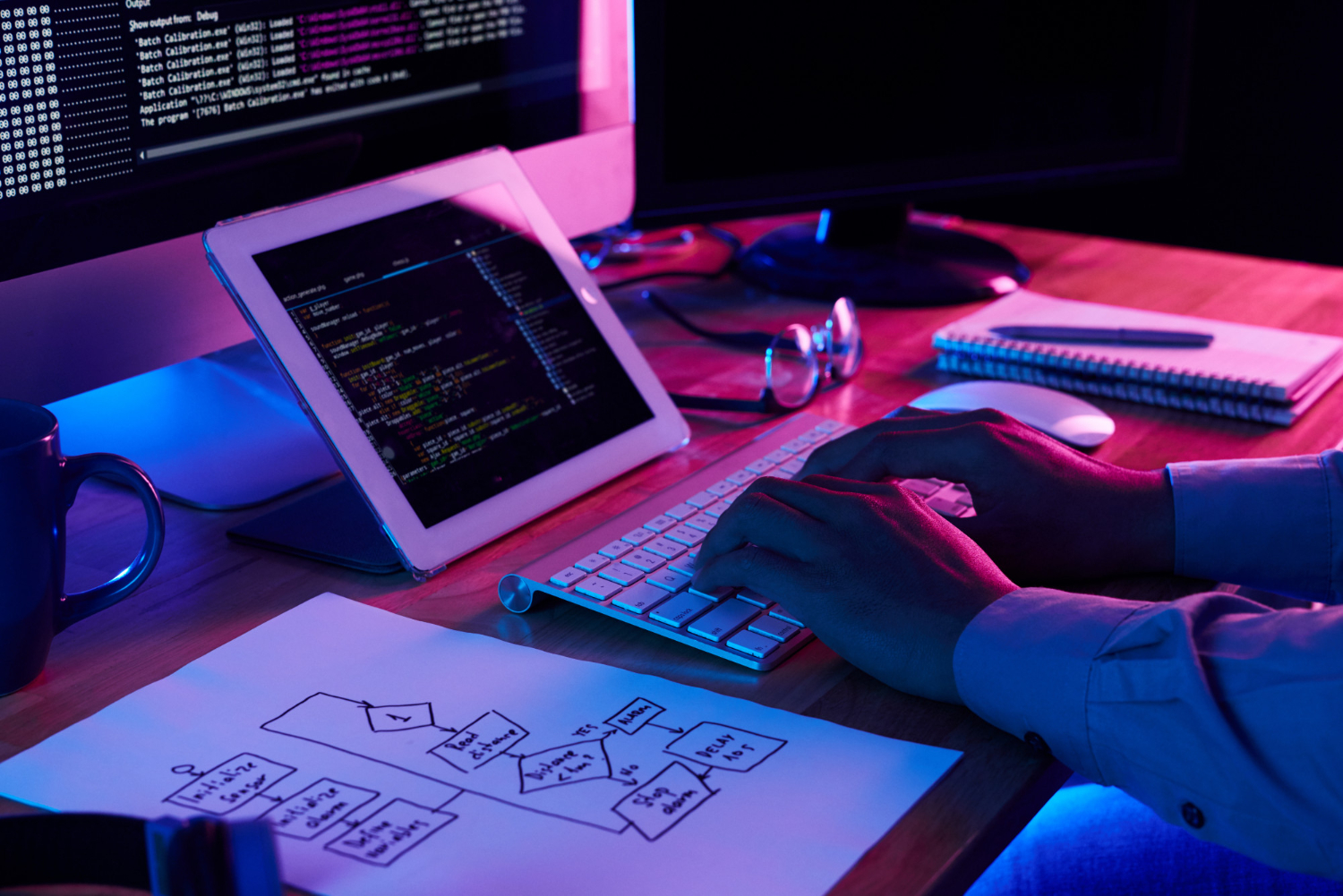
Python and Django Framework Online Training
Course Description
This comprehensive online course is designed to equip participants with the skills and knowledge needed for modern web development using Python and the Django framework. Covering both fundamental and advanced concepts, this course provides a hands-on approach to building web applications, from setting up the development environment to deploying a fully functional application.
Review each module to ensure a solid understanding, and join this course to start building your web application development skills today!
Module 1: Introduction to Python for Web Development
1.1 Introduction to Python
- Overview of Python programming language
- Basic syntax and data types
- Control flow and functions
1.2 Python Libraries for Web Development
- Introduction to popular Python libraries (e.g., Flask, Django)
- Understanding the role of libraries in web development
1.3 Setting Up the Development Environment
- Installing Python and virtual environments
- Configuring an integrated development environment (IDE)
Module 2: Introduction to Django Framework
2.1 Overview of Django
- Introduction to Django and its philosophy
- Comparison with other web frameworks
2.2 Django Project Setup
- Creating a new Django project
- Understanding project structure and configuration
2.3 Django Models and Databases
- Defining models and database tables
- Working with the Django ORM for database interactions
Module 3: Building Views and Templates
3.1 Django Views and URLs
- Creating views to handle HTTP requests
- Configuring URLs to map to views
3.2 Django Templates
- Understanding the Django templating engine
- Creating dynamic and reusable templates
3.3 Class-Based Views (CBVs)
- Introduction to Class-Based Views
- Using generic CBVs for common patterns
Module 4: Django Forms and User Authentication
4.1 Django Forms
- Creating forms for user input
- Form validation and handling
4.2 User Authentication and Authorization
- Implementing user registration and login functionality
- Managing user sessions and permissions
Module 5: Django Admin Interface and Middleware
5.1 Django Admin Interface
- Customizing the Django admin panel
- Managing models through the admin interface
5.2 Django Middleware
- Introduction to Django middleware
- Creating and using custom middleware components
Module 6: Django Rest Framework (Optional)
6.1 Introduction to Django Rest Framework (DRF)
- Building APIs with DRF
- Serializers and views for API development
6.2 Authentication and Permissions in DRF
- Configuring authentication in DRF
- Implementing permissions for API endpoints
Module 7: Testing and Debugging in Django
7.1 Unit Testing in Django
- Writing unit tests for Django applications
- Running tests and test suites
7.2 Debugging Django Applications
- Using debugging tools and techniques
- Handling common errors in Django development
Module 8: Deployment and Best Practices
8.1 Preparing for Deployment
- Configuring settings for production
- Setting up a production database
8.2 Deployment Platforms
- Deploying a Django application to platforms like Azure or AWS
- Best practices for securing and optimizing production environments